How the Move Programming Language Works
Table of Contents
.avif)
Move is a safe and powerful smart contract language used by Movement, Aptos, Sui, and other new blockchains. We’ll explain why its resource-oriented approach is so revolutionary, how Move prevents common vulnerabilities, and how the Move ecosystem will be linked to other chains thanks to Lumio L2.
What is Move?
Move is a secure and efficient smart contract programming language based on Rust. It powers blockchains like Aptos and Sui and offers many advantages compared to Solidity. Here at Pontem, we have used Move to create market-leading products like Pontem Wallet, Liquidswap DEX, and recently Lumio - a revolutionary L2 framework that supports all virtual machines, languages, and chains.
The origins of Move
Move was originally developed at Meta (the parent company of Facebook) as a proprietary smart contract language for its planned blockchain, Diem. Later, Move was made open-source, and now any developer can create dApps or even new blockchains with it.
Move is currently used by several blockchains, including Aptos, Sui, and Starcoin. Note that each of them has its own version of Move, though the core features are the same.
Move as a resource-oriented language
Before resources: object-oriented programming
In programming, an object is a package or structure that contains both data (also called variables, attributes or properties) and its behavior (functions or methods). For example, a dog can be coded as an object with attributes like size, weight, and color, and functions like run, bark, and wag the tail.
Once you’ve defined an object, you can move it around, reuse it as many times as you like in your program, make copies of it, or even destroy it as you want. Objects can also be combined in a modular fashion. Among the programming languages centered on objects are Java, Python, and C++. Rust, which served as a base for Move, also uses features of object-oriented programming.
Objects make it easier to create complex programs. For instance, if coding a game, you could code a health potion object once and then clone it a hundred times so that there’s a potion in every scene which then disappears forever once you open it. You can also have a magic tree that keeps producing new bottles of the potion.
The object model isn’t ideal for crypto assets, though, because a crypto token or an NFT has real monetary value. It shouldn’t be possible to clone it, re-use the same coin for two different transactions, store it in two different wallets at the same time, or accidentally destroy it.
Resources vs. objects
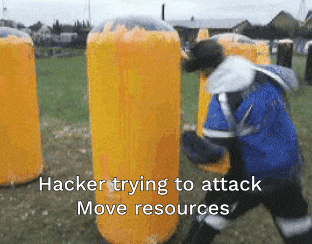
The creators of Move came up with a brilliant modification of the object concept: resources. A resource is a structure that has value, and there are some things that you can and cannot do with them:
- A resource can be moved, but it can’t be copied, implicitly discarded, or accidentally destroyed (though it can be destroyed on purpose by those with such access privileges). Once a structure is defined as a resource, it’s impossible to counterfeit it. You can only move a resource between different storage locations.
- Resource ownership is determined by storage location. If a token is stored in your account, you are the owner and you can send it, sell it, or whatever. If you don’t have it in the account, you can’t do anything with it. This is different from Ethereum, where you have to ask the blockchain ledger which user account is associated with a specific token, and ownership changes are registered on the main ledger as well.
- Resource functions (behavior, methods) can be triggered only by the owner. For example, if an NFT can be transferred, only its owner can trigger such a transaction.
- Most importantly, these rules are written into the code itself. They cannot be overridden by a malicious agent running a tweaked version of the virtual machine, for example.
To be fair, the developers of Move didn't invent the concept of resources. It comes from a mathematical logic system called linear logic, created by Jean-Yves Girad in 1987 which emphasizes formulas as resources, rather than complete truths or needing complete proofs. A resource in linear logic can only be used once, rather than treated as permanently true. (i.e. “If you give me A once, I’ll give you B once,” instead of “A can be exchanged for B.”) This makes linear logic useful in computer science, where confining a formula to a resource instead of treating it as a universal truth enables more versatile systems.
Move bytecode and Move VM
Move is a human-readable language, like all other smart contract languages. But in order for contract code to be executable by a machine, it has to be “translated” into a machine-readable format called bytecode. Here is an example of human-readable code in Java and an unrelated snippet of Java bytecode:
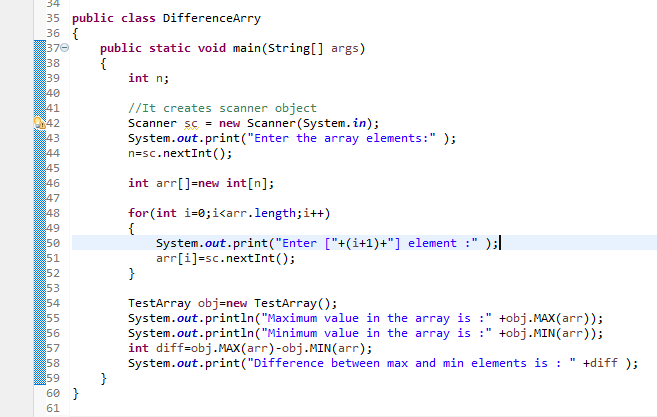
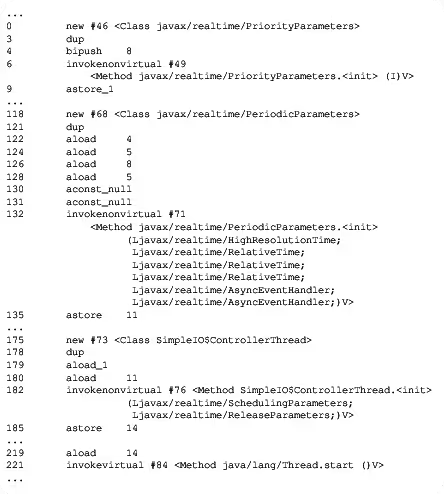
Languages are divided into two groups in terms of how their human-readable code is converted into bytecode: compiled and interpreted. In case of a compiled language, a tool called compiler first translates the code, and only after that it’s executed on-chain. Solidity is a compiled language, and the two tasks (compiling and execution) are handled by the EVM.
An interpreted language doesn’t need a compiler: instead, a bytecode interpreter reads and executes the code line by line in runtime. It can be slower than compiling, but it’s also more secure, because the interpreter also has debugging features, and you avoid introducing new errors at the compilation stage.
Strictly speaking, you can implement just about any language as both compiled or interpreted - it just depends on which tool the language creators choose to develop. So it’s more correct to speak of a certain language as typically compiled or typically interpreted.
Move is unusual in that it’s an interpreted language, but the Move on Aptos package includes a full set of tools for working with bytecode:
- Move Compiler v2 tool to translate contract code into bytecode;
- Bytecode verifier;
- Bytecode interpreter.
The result is increased security with much fewer runtime errors, combined with high execution speed. In general , which is then executed by Move Virtual Machine. Move VM is fast, secure, and resource-efficient - just like Move itself.
A word on blockchain virtual machines: generally each of them can only read the type of bytecode it’s built for, so you can’t plug a Move smart contract into EVM, for example. That’s why it’s so hard to migrate dApps between EVM and non-EVM blockchains: you have to rewrite all the code.
Read Pontem article on EVM and virtual machines
Luckily, there is a solution: Lumio, the first VM abstraction framework. It allows developers to deploy on any VM of their choice without reworking the code or worrying about compiling to the correct bytecode. Lumio is a federation of L2 chains that support Move VM, EVM, Solana VM (SVM), and so forth - and can settle on any L1 blockchain. For example, you can take a dApp written in Move and deploy it on EVM. Learn more about Lumio here.
Coming back to Move bytecode, there is a difference between how Move bytecode and EVM bytecode are executed. Move features a great tool called verifier, which checks new code for the safety of resources, types, and memory before it can be deployed. Unlike in an audit, these checks are done on-chain. Only after that a bytecode interpreter directly executes the code. This allows to reduce the number of runtime errors and security vulnerabilities.
For transparency’s sake, we should mention that in March 2023, the Zellic blockchain security firm discovered and fixed a critical bug in the bytecode verifier. If exploited, it could have allowed hackers to induce significant financial damage - for example, take out flash loans and not repay them. Needless to say, the issue was immediately fixed.
Formal verification in Move

Formal verification is a way to check a smart contract for vulnerabilities using a mathematical model, rather than code analysis and tests like in regular audits. The model in question is called a formal specification, and it describes how the contract should behave. Next, contract code itself is transformed into a set of mathematical statements, and, using a special tool called prover, one matches these statements to the formal specification.
The value of formal verification is that it can prove that a contract will behave properly regardless of the values of the variables that you plug into the formulas - a task that is impossible for a normal auditor. Also, you can uncover vulnerabilities that regular tests wouldn’t.
Read Pontem’s detailed guide to formal verification of smart contracts
Move is better adapted for formal verification than any other language, thanks to two features:
- Move Specification Language (MSL) - a modification of Move that can be easily translated to mathematical statements;
- Move Prover - an automated formal verification tool.
By the way, our Liquidswap DEX successfully passed formal verification by MoveBit - one of the few projects in the Move ecosystem to do so. It’s a very important step for Pontem, as it proved mathematicallymathematically that our smart contracts are free from vulnerabilities.
Parallel transaction execution and Move
Move is one of the few languages that supports parallel processing: blockchains like Aptos can handle many transactions simultaneously, instead of processing them sequentially the way Ethereum does. It’s thanks to this feature that Aptos can reach an incredible theoretical capacity of 160,000 TPS.
Transactions need to be independent from each other to be processed in parallel, and it’s up to each blockchain to build an execution engine that determines which is which. Aptos’ parallel processing engine is called Block-STM.
By the way, Rust is another language that enables parallel execution, and the Rust-based Solana also has this feature (though the architecture is very different).
Move as a modular language
Move code is organized into modules - vault-like units, each with its own name, that contain resources and the functions that each module allows. Outside code (scripts) can interact with the resources inside a module by calling only those functions that this specific module supports - which further adds to Move security.
The second main type of code unit in Move is a script - a snippet of code that is typically contained in a transaction. A script calls a module (or several) or initiates an action. The same transaction can feature several scripts and trigger several actions, which means you need fewer smart contracts for your dApp. This also adds to security (as each extra contract is a potential source of vulnerabilities) and improves user experience.
Note that Move being a modular smart contract language doesn’t make Move-based blockchains modular. Aptos isn’t a modular blockchain - and it doesn’t need to be, since it’s already extremely fast and cheap in its monolithic form. Celestia is an example of a modular chains, since it separates consensus, execution, and data availability - yet it supports contracts written in both modular Rust and non-modular Solidity.
Access control in Move
Access control is fundamental in blockchain: you have to make sure that a malicious third party cannot mint tokens, transfer them out of a contract, freeze or burn them, etc. A lot of dApp hacks become possible because of poor access control code in a contract.
In Move, assets represented as resources have access control built into them, because - like we said - the account that holds an asset also owns it. The trick is that if you send tokens to a smart contract, ownership doesn’t change. Move smart contracts can maintain information about the ownership and privileges of digital assets.
For instance, if a hacker manages to get access to a smart contract on Aptos, they won’t be able to withdraw any assets from it, unless the smart contract includes this functionality. This is because the assets held by the contract are owned by other accounts and only those accounts can move them out of the contract - a big security advantage over Solidity and Ethereum.
In other words, thanks to Move’s built-in access control, one user cannot transfer assets belonging to another. But since Move is also very flexible, you can customize the level and means of access to assets.
Move security
Let’s re-iterate the security advantages of Move over other smart contract languages:
- Prevents unauthorized access to assets (such as a hacker transferring tokens out of a contract and into their wallet) thanks to customizable access control;
- Prevents asset duplication and unintended destruction, plus common vulnerabilities like double-spending, thanks to the atomic resource structure - assets being represented as resources that can’t be copied or destroyed;
- Prevents common contract code errors thanks to the on-chain bytecode verifier that obligatorily checks all new code before it can be deployed;
- Supports formal verification out of the box thanks to Move Prover;
- Allows code external to a module to call only the functions allowed by the module (i.e. an external script cannot trigger any malicious action);
- Minimizes the number of contracts required by an app by allowing a single transaction to contain several scripts and call several actions.
Move and Solidity: how Pontem builds links between the languages
Move and Move-powered virtual machines are very different from Solidity and EVM, or from SVM and Solana. You can’t take an Ethereum app written in Solidity and port it into the ecosystem of Aptos or Sui. There are two solutions, however:
- Lumio L2: the first VM abstraction framework, built by Pontem. It allows devs to deploy dApps on any VM without rewriting code. For example, you can take a Move app and deploy it on an instance of Lumio that supports Move VM but settles on Ethereum, thus integrating it into the Ethereum ecosystem. No code rewrites are needed.
- ByteBabel: also by Pontem, this is an industry-first Solidity-to-Move code transpiler. We’ve addressed foundational challenges and advanced on complex issues, though dynamic dispatch and delegate calls are still challenging. Now, we’re open-sourcing it for the public: GitHub.
In conclusion
Move is all-round awesome - fast, safe, resource-efficient, flexible, and surprisingly easy to learn. We’re convinced that the Move ecosystem is poised for explosive growth in the next few years - and one of our main goals in Pontem is to link it with the wider blockchain space. We are making bigger strides in this direction than anyone in the space - and we hope that more and more developers will enter the universe of Move with our assistance.
About Pontem
Pontem Network is a product studio building foundational dApps for the Move ecosystem. Our products include:
- Pontem Wallet -- the best Aptos wallet
- Lumio L2 - the first VM-agnostic L2 framework
- Liquidswap -- the first DEX (AMM) for Aptos
- $LSD - the official token of Liquidswap DAO
- Move Playground -- a simple browser Move code editor
- Move IntelliJ IDE plugin for developers